- Clean up
- Removed notes - Updated readme - DB - Updated initial db schema - Updated db seed - Created source, station, and tag models - Libs - Create content source importer - UI - Added content source UI & routes - Updated page layout - Created <Breadcrumbs> component
This commit is contained in:
parent
560c853d33
commit
4e0dc08e29
179
README.md
179
README.md
@ -1,180 +1,15 @@
|
||||
# Remix Indie Stack
|
||||
|
||||
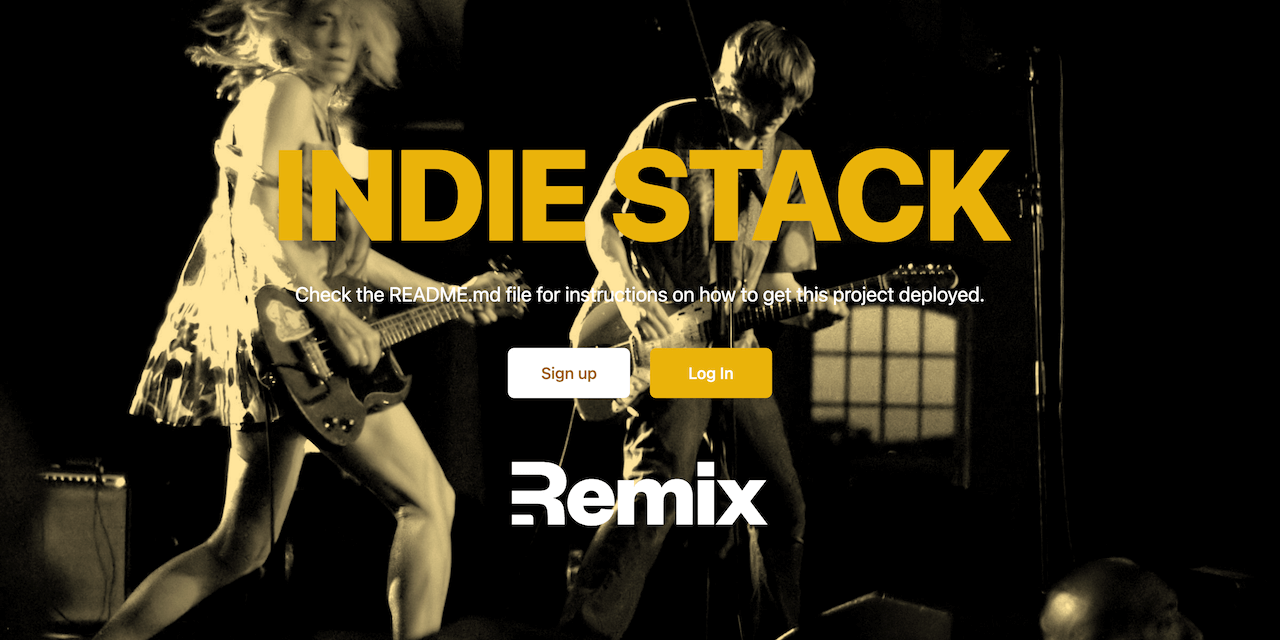
|
||||
|
||||
Learn more about [Remix Stacks](https://remix.run/stacks).
|
||||
|
||||
```sh
|
||||
npx create-remix@latest --template remix-run/indie-stack
|
||||
```
|
||||
|
||||
## What's in the stack
|
||||
|
||||
- [Fly app deployment](https://fly.io) with [Docker](https://www.docker.com/)
|
||||
- Production-ready [SQLite Database](https://sqlite.org)
|
||||
- Healthcheck endpoint for [Fly backups region fallbacks](https://fly.io/docs/reference/configuration/#services-http_checks)
|
||||
- [GitHub Actions](https://github.com/features/actions) for deploy on merge to production and staging environments
|
||||
- Email/Password Authentication with [cookie-based sessions](https://remix.run/utils/sessions#md-createcookiesessionstorage)
|
||||
- Database ORM with [Prisma](https://prisma.io)
|
||||
- Styling with [Tailwind](https://tailwindcss.com/)
|
||||
- End-to-end testing with [Cypress](https://cypress.io)
|
||||
- Local third party request mocking with [MSW](https://mswjs.io)
|
||||
- Unit testing with [Vitest](https://vitest.dev) and [Testing Library](https://testing-library.com)
|
||||
- Code formatting with [Prettier](https://prettier.io)
|
||||
- Linting with [ESLint](https://eslint.org)
|
||||
- Static Types with [TypeScript](https://typescriptlang.org)
|
||||
|
||||
Not a fan of bits of the stack? Fork it, change it, and use `npx create-remix --template your/repo`! Make it your own.
|
||||
|
||||
## Quickstart
|
||||
|
||||
Click this button to create a [Gitpod](https://gitpod.io) workspace with the project set up and Fly pre-installed
|
||||
|
||||
[](https://gitpod.io/#https://github.com/remix-run/indie-stack/tree/main)
|
||||
# Awesome Radio
|
||||
|
||||
## Development
|
||||
|
||||
- This step only applies if you've opted out of having the CLI install dependencies for you:
|
||||
### Running
|
||||
|
||||
```sh
|
||||
npx remix init
|
||||
```
|
||||
|
||||
- Initial setup: _If you just generated this project, this step has been done for you._
|
||||
|
||||
```sh
|
||||
npm run setup
|
||||
```
|
||||
|
||||
- Start dev server:
|
||||
|
||||
```sh
|
||||
npm run dev
|
||||
```
|
||||
|
||||
This starts your app in development mode, rebuilding assets on file changes.
|
||||
|
||||
The database seed script creates a new user with some data you can use to get started:
|
||||
|
||||
- Email: `rachel@remix.run`
|
||||
- Password: `racheliscool`
|
||||
|
||||
### Relevant code:
|
||||
|
||||
This is a pretty simple note-taking app, but it's a good example of how you can build a full stack app with Prisma and Remix. The main functionality is creating users, logging in and out, and creating and deleting notes.
|
||||
|
||||
- creating users, and logging in and out [./app/models/user.server.ts](./app/models/user.server.ts)
|
||||
- user sessions, and verifying them [./app/session.server.ts](./app/session.server.ts)
|
||||
- creating, and deleting notes [./app/models/note.server.ts](./app/models/note.server.ts)
|
||||
|
||||
## Deployment
|
||||
|
||||
This Remix Stack comes with two GitHub Actions that handle automatically deploying your app to production and staging environments.
|
||||
|
||||
Prior to your first deployment, you'll need to do a few things:
|
||||
|
||||
- [Install Fly](https://fly.io/docs/getting-started/installing-flyctl/)
|
||||
|
||||
- Sign up and log in to Fly
|
||||
|
||||
```sh
|
||||
fly auth signup
|
||||
```
|
||||
|
||||
> **Note:** If you have more than one Fly account, ensure that you are signed into the same account in the Fly CLI as you are in the browser. In your terminal, run `fly auth whoami` and ensure the email matches the Fly account signed into the browser.
|
||||
|
||||
- Create two apps on Fly, one for staging and one for production:
|
||||
|
||||
```sh
|
||||
fly apps create tunein-radio-stations-1ae3
|
||||
fly apps create tunein-radio-stations-1ae3-staging
|
||||
```
|
||||
|
||||
> **Note:** Make sure this name matches the `app` set in your `fly.toml` file. Otherwise, you will not be able to deploy.
|
||||
|
||||
- Initialize Git.
|
||||
|
||||
```sh
|
||||
git init
|
||||
```
|
||||
|
||||
- Create a new [GitHub Repository](https://repo.new), and then add it as the remote for your project. **Do not push your app yet!**
|
||||
|
||||
```sh
|
||||
git remote add origin <ORIGIN_URL>
|
||||
```
|
||||
|
||||
- Add a `FLY_API_TOKEN` to your GitHub repo. To do this, go to your user settings on Fly and create a new [token](https://web.fly.io/user/personal_access_tokens/new), then add it to [your repo secrets](https://docs.github.com/en/actions/security-guides/encrypted-secrets) with the name `FLY_API_TOKEN`.
|
||||
|
||||
- Add a `SESSION_SECRET` to your fly app secrets, to do this you can run the following commands:
|
||||
|
||||
```sh
|
||||
fly secrets set SESSION_SECRET=$(openssl rand -hex 32) --app tunein-radio-stations-1ae3
|
||||
fly secrets set SESSION_SECRET=$(openssl rand -hex 32) --app tunein-radio-stations-1ae3-staging
|
||||
```
|
||||
|
||||
If you don't have openssl installed, you can also use [1Password](https://1password.com/password-generator) to generate a random secret, just replace `$(openssl rand -hex 32)` with the generated secret.
|
||||
|
||||
- Create a persistent volume for the sqlite database for both your staging and production environments. Run the following:
|
||||
|
||||
```sh
|
||||
fly volumes create data --size 1 --app tunein-radio-stations-1ae3
|
||||
fly volumes create data --size 1 --app tunein-radio-stations-1ae3-staging
|
||||
```
|
||||
|
||||
Now that everything is set up you can commit and push your changes to your repo. Every commit to your `main` branch will trigger a deployment to your production environment, and every commit to your `dev` branch will trigger a deployment to your staging environment.
|
||||
|
||||
### Connecting to your database
|
||||
|
||||
The sqlite database lives at `/data/sqlite.db` in your deployed application. You can connect to the live database by running `fly ssh console -C database-cli`.
|
||||
|
||||
### Getting Help with Deployment
|
||||
|
||||
If you run into any issues deploying to Fly, make sure you've followed all of the steps above and if you have, then post as many details about your deployment (including your app name) to [the Fly support community](https://community.fly.io). They're normally pretty responsive over there and hopefully can help resolve any of your deployment issues and questions.
|
||||
|
||||
## GitHub Actions
|
||||
|
||||
We use GitHub Actions for continuous integration and deployment. Anything that gets into the `main` branch will be deployed to production after running tests/build/etc. Anything in the `dev` branch will be deployed to staging.
|
||||
|
||||
## Testing
|
||||
|
||||
### Cypress
|
||||
|
||||
We use Cypress for our End-to-End tests in this project. You'll find those in the `cypress` directory. As you make changes, add to an existing file or create a new file in the `cypress/e2e` directory to test your changes.
|
||||
|
||||
We use [`@testing-library/cypress`](https://testing-library.com/cypress) for selecting elements on the page semantically.
|
||||
|
||||
To run these tests in development, run `npm run test:e2e:dev` which will start the dev server for the app as well as the Cypress client. Make sure the database is running in docker as described above.
|
||||
|
||||
We have a utility for testing authenticated features without having to go through the login flow:
|
||||
|
||||
```ts
|
||||
cy.login();
|
||||
// you are now logged in as a new user
|
||||
```shell
|
||||
npm run dev
|
||||
```
|
||||
|
||||
We also have a utility to auto-delete the user at the end of your test. Just make sure to add this in each test file:
|
||||
### Testing
|
||||
|
||||
```ts
|
||||
afterEach(() => {
|
||||
cy.cleanupUser();
|
||||
});
|
||||
```shell
|
||||
npm run test
|
||||
```
|
||||
|
||||
That way, we can keep your local db clean and keep your tests isolated from one another.
|
||||
|
||||
### Vitest
|
||||
|
||||
For lower level tests of utilities and individual components, we use `vitest`. We have DOM-specific assertion helpers via [`@testing-library/jest-dom`](https://testing-library.com/jest-dom).
|
||||
|
||||
### Type Checking
|
||||
|
||||
This project uses TypeScript. It's recommended to get TypeScript set up for your editor to get a really great in-editor experience with type checking and auto-complete. To run type checking across the whole project, run `npm run typecheck`.
|
||||
|
||||
### Linting
|
||||
|
||||
This project uses ESLint for linting. That is configured in `.eslintrc.js`.
|
||||
|
||||
### Formatting
|
||||
|
||||
We use [Prettier](https://prettier.io/) for auto-formatting in this project. It's recommended to install an editor plugin (like the [VSCode Prettier plugin](https://marketplace.visualstudio.com/items?itemName=esbenp.prettier-vscode)) to get auto-formatting on save. There's also a `npm run format` script you can run to format all files in the project.
|
||||
|
18
app/components/breadcrumbs.tsx
Normal file
18
app/components/breadcrumbs.tsx
Normal file
@ -0,0 +1,18 @@
|
||||
import type { ReactNode } from "react";
|
||||
|
||||
export type BreadcrumbsProps = {
|
||||
children: ReactNode | ReactNode[];
|
||||
}
|
||||
|
||||
export function Breadcrumbs({ children }: BreadcrumbsProps) {
|
||||
const links = Array.isArray(children) ? children : [children];
|
||||
return (
|
||||
<div className="text-lg breadcrumbs">
|
||||
<ul>
|
||||
{links.map((c, i) => {
|
||||
return <li key={i}>{c}</li>;
|
||||
})}
|
||||
</ul>
|
||||
</div>
|
||||
);
|
||||
}
|
@ -1,4 +1,5 @@
|
||||
import { RadioIcon } from "@heroicons/react/24/solid";
|
||||
import { NavLink } from "@remix-run/react";
|
||||
import type { ReactNode } from "react";
|
||||
|
||||
export type PageLayoutProps = {
|
||||
@ -31,19 +32,35 @@ export function PageLayout({ children }: PageLayoutProps) {
|
||||
</ul>
|
||||
</div>
|
||||
</div>
|
||||
|
||||
|
||||
{children}
|
||||
<div className="py-2 px-6">
|
||||
{children}
|
||||
</div>
|
||||
</div>
|
||||
<div className="drawer-side">
|
||||
<label htmlFor="primary-drawer" className="drawer-overlay"></label>
|
||||
<ul className="menu menu-compact flex w-80 flex-col bg-base-200 text-base-content p-0 px-4 pt-4">
|
||||
<li className="flex flex-row justify-start gap-2 ">
|
||||
<li className="flex flex-row justify-start gap-2 mb-4">
|
||||
<RadioIcon className="h-8 w-8 p-0" />
|
||||
<h1 className="text-2xl p-0">Awesome Radio</h1>
|
||||
</li>
|
||||
<li><a>Sidebar Item 1</a></li>
|
||||
<li><a>Sidebar Item 2</a></li>
|
||||
<li className="menu-title">
|
||||
<span>Listen</span>
|
||||
</li>
|
||||
<li>
|
||||
<NavLink to="/listen/music">Music</NavLink>
|
||||
</li>
|
||||
<li>
|
||||
<NavLink to="/listen/sports">Sports</NavLink>
|
||||
</li>
|
||||
<li>
|
||||
<NavLink to="/listen/news">News & Talk</NavLink>
|
||||
</li>
|
||||
<li className="menu-title">
|
||||
<span>Manage Content</span>
|
||||
</li>
|
||||
<li>
|
||||
<NavLink to="/sources">Sources</NavLink>
|
||||
</li>
|
||||
|
||||
</ul>
|
||||
|
||||
|
@ -1,9 +1,32 @@
|
||||
import { PrismaClient } from "@prisma/client";
|
||||
|
||||
function createPrismaClient() {
|
||||
return new PrismaClient({
|
||||
// log: [
|
||||
// {
|
||||
// emit: "stdout",
|
||||
// level: "query"
|
||||
// },
|
||||
// {
|
||||
// emit: "stdout",
|
||||
// level: "error"
|
||||
// },
|
||||
// {
|
||||
// emit: "stdout",
|
||||
// level: "info"
|
||||
// },
|
||||
// {
|
||||
// emit: "stdout",
|
||||
// level: "warn"
|
||||
// }
|
||||
// ]
|
||||
});
|
||||
}
|
||||
|
||||
let prisma: PrismaClient;
|
||||
|
||||
declare global {
|
||||
var __db__: PrismaClient | undefined;
|
||||
var __db__: PrismaClient | undefined;
|
||||
}
|
||||
|
||||
// This is needed because in development we don't want to restart
|
||||
@ -11,13 +34,13 @@ declare global {
|
||||
// create a new connection to the DB with every change either.
|
||||
// In production, we'll have a single connection to the DB.
|
||||
if (process.env.NODE_ENV === "production") {
|
||||
prisma = new PrismaClient();
|
||||
prisma = createPrismaClient();
|
||||
} else {
|
||||
if (!global.__db__) {
|
||||
global.__db__ = new PrismaClient();
|
||||
}
|
||||
prisma = global.__db__;
|
||||
prisma.$connect();
|
||||
if (!global.__db__) {
|
||||
global.__db__ = createPrismaClient();
|
||||
}
|
||||
prisma = global.__db__;
|
||||
prisma.$connect();
|
||||
}
|
||||
|
||||
export { prisma };
|
||||
|
24
app/lib/importer.server.ts
Normal file
24
app/lib/importer.server.ts
Normal file
@ -0,0 +1,24 @@
|
||||
import type { ContentSource } from "@prisma/client";
|
||||
import { fetch } from "@remix-run/node";
|
||||
import { importStations } from "~/models/station.server";
|
||||
|
||||
|
||||
export async function importSource(source: ContentSource) {
|
||||
switch (source.type) {
|
||||
case "json":
|
||||
return importRemoteJson(source);
|
||||
default:
|
||||
throw new Error("Unsupported source");
|
||||
|
||||
}
|
||||
}
|
||||
|
||||
export async function importRemoteJson(source: ContentSource) {
|
||||
const response = await fetch(source.connectionUrl);
|
||||
if (!response.ok) {
|
||||
throw new Error("Failed to fetch source");
|
||||
}
|
||||
const { data } = await response.json();
|
||||
return importStations(data);
|
||||
|
||||
}
|
@ -1,52 +0,0 @@
|
||||
import type { User, Note } from "@prisma/client";
|
||||
|
||||
import { prisma } from "~/db.server";
|
||||
|
||||
export function getNote({
|
||||
id,
|
||||
userId,
|
||||
}: Pick<Note, "id"> & {
|
||||
userId: User["id"];
|
||||
}) {
|
||||
return prisma.note.findFirst({
|
||||
select: { id: true, body: true, title: true },
|
||||
where: { id, userId },
|
||||
});
|
||||
}
|
||||
|
||||
export function getNoteListItems({ userId }: { userId: User["id"] }) {
|
||||
return prisma.note.findMany({
|
||||
where: { userId },
|
||||
select: { id: true, title: true },
|
||||
orderBy: { updatedAt: "desc" },
|
||||
});
|
||||
}
|
||||
|
||||
export function createNote({
|
||||
body,
|
||||
title,
|
||||
userId,
|
||||
}: Pick<Note, "body" | "title"> & {
|
||||
userId: User["id"];
|
||||
}) {
|
||||
return prisma.note.create({
|
||||
data: {
|
||||
title,
|
||||
body,
|
||||
user: {
|
||||
connect: {
|
||||
id: userId,
|
||||
},
|
||||
},
|
||||
},
|
||||
});
|
||||
}
|
||||
|
||||
export function deleteNote({
|
||||
id,
|
||||
userId,
|
||||
}: Pick<Note, "id"> & { userId: User["id"] }) {
|
||||
return prisma.note.deleteMany({
|
||||
where: { id, userId },
|
||||
});
|
||||
}
|
34
app/models/source.server.ts
Normal file
34
app/models/source.server.ts
Normal file
@ -0,0 +1,34 @@
|
||||
import { prisma } from "~/db.server";
|
||||
|
||||
export type SourceInput = {
|
||||
id?: string;
|
||||
name: string;
|
||||
description?: string;
|
||||
connectionUrl: string;
|
||||
type: string;
|
||||
|
||||
};
|
||||
|
||||
export function saveSource({ id, name, description, connectionUrl, type }: SourceInput) {
|
||||
const data = { name, description, connectionUrl, type };
|
||||
if (id) {
|
||||
return prisma.contentSource.update({
|
||||
where: { id },
|
||||
data
|
||||
});
|
||||
}
|
||||
return prisma.contentSource.create({
|
||||
data
|
||||
});
|
||||
}
|
||||
|
||||
|
||||
export function getSources() {
|
||||
return prisma.contentSource.findMany({
|
||||
orderBy: [{ name: "asc" }]
|
||||
});
|
||||
}
|
||||
|
||||
export function getSource(id: string) {
|
||||
return prisma.contentSource.findUnique({ where: { id } });
|
||||
}
|
71
app/models/station.server.ts
Normal file
71
app/models/station.server.ts
Normal file
@ -0,0 +1,71 @@
|
||||
import type { PrismaClient, Station, Tag } from "@prisma/client";
|
||||
import { prisma } from "~/db.server";
|
||||
import { upsertTagOnName } from "~/models/tag.server";
|
||||
import { slugify } from "~/utils";
|
||||
|
||||
export type StationInput = {
|
||||
id?: string
|
||||
name: string;
|
||||
description: string;
|
||||
streamUrl: string;
|
||||
imgUrl: string;
|
||||
reliability: number;
|
||||
popularity: number;
|
||||
tags: string[]
|
||||
};
|
||||
|
||||
export type PrismaTxClient = Omit<PrismaClient, "$connect" | "$disconnect" | "$on" | "$transaction" | "$use">;
|
||||
|
||||
export function upsertStationOnStreamUrl(input: StationInput, p: PrismaTxClient = prisma) {
|
||||
return p.station.upsert({
|
||||
where: { streamUrl: input.streamUrl },
|
||||
create: {
|
||||
name: input.name,
|
||||
slug: slugify(input.name),
|
||||
description: input.description,
|
||||
imgUrl: input.imgUrl,
|
||||
streamUrl: input.streamUrl,
|
||||
reliability: input.reliability,
|
||||
popularity: input.popularity
|
||||
},
|
||||
update: {
|
||||
name: input.name,
|
||||
slug: slugify(input.name),
|
||||
description: input.description,
|
||||
imgUrl: input.imgUrl,
|
||||
reliability: input.reliability,
|
||||
popularity: input.popularity
|
||||
}
|
||||
});
|
||||
}
|
||||
|
||||
export function deleteStationTags(station: Station, p: PrismaTxClient = prisma) {
|
||||
return p.stationTag.deleteMany({ where: { stationId: station.id } });
|
||||
}
|
||||
|
||||
export function createStationTag(station: Station, tag: Tag, p: PrismaTxClient = prisma) {
|
||||
return p.stationTag.create({
|
||||
data: {
|
||||
tagId: tag.id,
|
||||
stationId: station.id
|
||||
}
|
||||
});
|
||||
}
|
||||
|
||||
export async function importStations(stationsInput: StationInput[]) {
|
||||
return prisma.$transaction(async (tx) => {
|
||||
const stations: Station[] = [];
|
||||
for (const stationInput of stationsInput) {
|
||||
const station = await upsertStationOnStreamUrl(stationInput, tx);
|
||||
await deleteStationTags(station, tx);
|
||||
for (const tagName of stationInput.tags) {
|
||||
const tag = await upsertTagOnName(tagName, tx);
|
||||
await createStationTag(station, tag, tx);
|
||||
}
|
||||
stations.push(station);
|
||||
}
|
||||
return stations;
|
||||
});
|
||||
|
||||
|
||||
}
|
12
app/models/tag.server.ts
Normal file
12
app/models/tag.server.ts
Normal file
@ -0,0 +1,12 @@
|
||||
import { prisma } from "~/db.server";
|
||||
import type { PrismaTxClient } from "~/models/station.server";
|
||||
import { slugify } from "~/utils";
|
||||
|
||||
export function upsertTagOnName(tag: string, p: PrismaTxClient = prisma) {
|
||||
const slug = slugify(tag);
|
||||
return p.tag.upsert({
|
||||
where: { name: tag },
|
||||
create: { name: tag, slug },
|
||||
update: { slug }
|
||||
});
|
||||
}
|
52
app/root.tsx
52
app/root.tsx
@ -1,7 +1,16 @@
|
||||
import { cssBundleHref } from "@remix-run/css-bundle";
|
||||
import type { LinksFunction, LoaderArgs } from "@remix-run/node";
|
||||
import { json } from "@remix-run/node";
|
||||
import { Links, LiveReload, Meta, Outlet, Scripts, ScrollRestoration } from "@remix-run/react";
|
||||
import {
|
||||
isRouteErrorResponse,
|
||||
Links,
|
||||
LiveReload,
|
||||
Meta,
|
||||
Outlet,
|
||||
Scripts,
|
||||
ScrollRestoration,
|
||||
useRouteError
|
||||
} from "@remix-run/react";
|
||||
import type { ReactNode } from "react";
|
||||
import { PageLayout } from "~/components/page-layout";
|
||||
|
||||
@ -53,12 +62,49 @@ export default function App() {
|
||||
);
|
||||
}
|
||||
|
||||
export function ErrorBoundary({ error }: { error: Error }) {
|
||||
export function ErrorBoundary() {
|
||||
const error = useRouteError();
|
||||
|
||||
console.error(error);
|
||||
|
||||
if (isRouteErrorResponse(error)) {
|
||||
const title = `${error.status} - ${error.statusText}`;
|
||||
return (
|
||||
<Document title={title}>
|
||||
<div className="container mx-auto p-4 prose w-full">
|
||||
<h1>{title}</h1>
|
||||
</div>
|
||||
</Document>
|
||||
);
|
||||
}
|
||||
|
||||
if (error instanceof Error) {
|
||||
return (
|
||||
<Document title="Uh-oh!">
|
||||
<div className="container mx-auto p-4 prose w-full">
|
||||
<h2>Yikes!</h2>
|
||||
<div className="alert alert-error shadow-lg">
|
||||
<div>
|
||||
<svg xmlns="http://www.w3.org/2000/svg" className="stroke-current flex-shrink-0 h-6 w-6"
|
||||
fill="none" viewBox="0 0 24 24">
|
||||
<path strokeLinecap="round" strokeLinejoin="round" strokeWidth="2"
|
||||
d="M10 14l2-2m0 0l2-2m-2 2l-2-2m2 2l2 2m7-2a9 9 0 11-18 0 9 9 0 0118 0z" />
|
||||
</svg>
|
||||
<span>{error.message}</span>
|
||||
</div>
|
||||
</div>
|
||||
<h2>Stack Trace</h2>
|
||||
<div className="mockup-code">
|
||||
<pre data-prefix="!!!"><code>{error.stack}</code></pre>
|
||||
</div>
|
||||
</div>
|
||||
</Document>
|
||||
);
|
||||
}
|
||||
|
||||
return (
|
||||
<Document title="Uh-oh!">
|
||||
<div className="flex min-h-screen flex-1 flex-col items-center justify-center py-4">
|
||||
<div className="container mx-auto p-4 prose w-full">
|
||||
<h1>Sorry, something went wrong...</h1>
|
||||
</div>
|
||||
</Document>
|
||||
|
@ -2,7 +2,7 @@ import type { V2_MetaFunction } from "@remix-run/node";
|
||||
|
||||
import { useOptionalUser } from "~/utils";
|
||||
|
||||
export const meta: V2_MetaFunction = () => [{ title: "Remix Notes" }];
|
||||
export const meta: V2_MetaFunction = () => [{ title: "Awesome Radio" }];
|
||||
|
||||
export default function Index() {
|
||||
const user = useOptionalUser();
|
||||
|
@ -62,8 +62,8 @@ export const meta: V2_MetaFunction = () => [{ title: "Login" }];
|
||||
|
||||
export default function LoginPage() {
|
||||
const [searchParams] = useSearchParams();
|
||||
const redirectTo = searchParams.get("redirectTo") || "/notes";
|
||||
const actionData = useActionData<typeof action>();
|
||||
const redirectTo = searchParams.get("redirectTo") || "/";
|
||||
const actionData = useActionData<typeof action>();
|
||||
const emailRef = useRef<HTMLInputElement>(null);
|
||||
const passwordRef = useRef<HTMLInputElement>(null);
|
||||
|
||||
|
@ -1,70 +0,0 @@
|
||||
import type { ActionArgs, LoaderArgs } from "@remix-run/node";
|
||||
import { json, redirect } from "@remix-run/node";
|
||||
import {
|
||||
Form,
|
||||
isRouteErrorResponse,
|
||||
useLoaderData,
|
||||
useRouteError,
|
||||
} from "@remix-run/react";
|
||||
import invariant from "tiny-invariant";
|
||||
|
||||
import { deleteNote, getNote } from "~/models/note.server";
|
||||
import { requireUserId } from "~/session.server";
|
||||
|
||||
export const loader = async ({ params, request }: LoaderArgs) => {
|
||||
const userId = await requireUserId(request);
|
||||
invariant(params.noteId, "noteId not found");
|
||||
|
||||
const note = await getNote({ id: params.noteId, userId });
|
||||
if (!note) {
|
||||
throw new Response("Not Found", { status: 404 });
|
||||
}
|
||||
return json({ note });
|
||||
};
|
||||
|
||||
export const action = async ({ params, request }: ActionArgs) => {
|
||||
const userId = await requireUserId(request);
|
||||
invariant(params.noteId, "noteId not found");
|
||||
|
||||
await deleteNote({ id: params.noteId, userId });
|
||||
|
||||
return redirect("/notes");
|
||||
};
|
||||
|
||||
export default function NoteDetailsPage() {
|
||||
const data = useLoaderData<typeof loader>();
|
||||
|
||||
return (
|
||||
<div>
|
||||
<h3 className="text-2xl font-bold">{data.note.title}</h3>
|
||||
<p className="py-6">{data.note.body}</p>
|
||||
<hr className="my-4" />
|
||||
<Form method="post">
|
||||
<button
|
||||
type="submit"
|
||||
className="rounded bg-blue-500 px-4 py-2 text-white hover:bg-blue-600 focus:bg-blue-400"
|
||||
>
|
||||
Delete
|
||||
</button>
|
||||
</Form>
|
||||
</div>
|
||||
);
|
||||
}
|
||||
|
||||
export function ErrorBoundary() {
|
||||
const error = useRouteError();
|
||||
|
||||
if (error instanceof Error) {
|
||||
return <div>An unexpected error occurred: {error.message}</div>;
|
||||
}
|
||||
|
||||
if (!isRouteErrorResponse(error)) {
|
||||
return <h1>Unknown Error</h1>;
|
||||
}
|
||||
|
||||
if (error.status === 404) {
|
||||
return <div>Note not found</div>;
|
||||
}
|
||||
|
||||
return <div>An unexpected error occurred: {error.statusText}</div>;
|
||||
}
|
@ -1,12 +0,0 @@
|
||||
import { Link } from "@remix-run/react";
|
||||
|
||||
export default function NoteIndexPage() {
|
||||
return (
|
||||
<p>
|
||||
No note selected. Select a note on the left, or{" "}
|
||||
<Link to="new" className="text-blue-500 underline">
|
||||
create a new note.
|
||||
</Link>
|
||||
</p>
|
||||
);
|
||||
}
|
@ -1,109 +0,0 @@
|
||||
import type { ActionArgs } from "@remix-run/node";
|
||||
import { json, redirect } from "@remix-run/node";
|
||||
import { Form, useActionData } from "@remix-run/react";
|
||||
import { useEffect, useRef } from "react";
|
||||
|
||||
import { createNote } from "~/models/note.server";
|
||||
import { requireUserId } from "~/session.server";
|
||||
|
||||
export const action = async ({ request }: ActionArgs) => {
|
||||
const userId = await requireUserId(request);
|
||||
|
||||
const formData = await request.formData();
|
||||
const title = formData.get("title");
|
||||
const body = formData.get("body");
|
||||
|
||||
if (typeof title !== "string" || title.length === 0) {
|
||||
return json(
|
||||
{ errors: { body: null, title: "Title is required" } },
|
||||
{ status: 400 }
|
||||
);
|
||||
}
|
||||
|
||||
if (typeof body !== "string" || body.length === 0) {
|
||||
return json(
|
||||
{ errors: { body: "Body is required", title: null } },
|
||||
{ status: 400 }
|
||||
);
|
||||
}
|
||||
|
||||
const note = await createNote({ body, title, userId });
|
||||
|
||||
return redirect(`/notes/${note.id}`);
|
||||
};
|
||||
|
||||
export default function NewNotePage() {
|
||||
const actionData = useActionData<typeof action>();
|
||||
const titleRef = useRef<HTMLInputElement>(null);
|
||||
const bodyRef = useRef<HTMLTextAreaElement>(null);
|
||||
|
||||
useEffect(() => {
|
||||
if (actionData?.errors?.title) {
|
||||
titleRef.current?.focus();
|
||||
} else if (actionData?.errors?.body) {
|
||||
bodyRef.current?.focus();
|
||||
}
|
||||
}, [actionData]);
|
||||
|
||||
return (
|
||||
<Form
|
||||
method="post"
|
||||
style={{
|
||||
display: "flex",
|
||||
flexDirection: "column",
|
||||
gap: 8,
|
||||
width: "100%",
|
||||
}}
|
||||
>
|
||||
<div>
|
||||
<label className="flex w-full flex-col gap-1">
|
||||
<span>Title: </span>
|
||||
<input
|
||||
ref={titleRef}
|
||||
name="title"
|
||||
className="flex-1 rounded-md border-2 border-blue-500 px-3 text-lg leading-loose"
|
||||
aria-invalid={actionData?.errors?.title ? true : undefined}
|
||||
aria-errormessage={
|
||||
actionData?.errors?.title ? "title-error" : undefined
|
||||
}
|
||||
/>
|
||||
</label>
|
||||
{actionData?.errors?.title ? (
|
||||
<div className="pt-1 text-red-700" id="title-error">
|
||||
{actionData.errors.title}
|
||||
</div>
|
||||
) : null}
|
||||
</div>
|
||||
|
||||
<div>
|
||||
<label className="flex w-full flex-col gap-1">
|
||||
<span>Body: </span>
|
||||
<textarea
|
||||
ref={bodyRef}
|
||||
name="body"
|
||||
rows={8}
|
||||
className="w-full flex-1 rounded-md border-2 border-blue-500 px-3 py-2 text-lg leading-6"
|
||||
aria-invalid={actionData?.errors?.body ? true : undefined}
|
||||
aria-errormessage={
|
||||
actionData?.errors?.body ? "body-error" : undefined
|
||||
}
|
||||
/>
|
||||
</label>
|
||||
{actionData?.errors?.body ? (
|
||||
<div className="pt-1 text-red-700" id="body-error">
|
||||
{actionData.errors.body}
|
||||
</div>
|
||||
) : null}
|
||||
</div>
|
||||
|
||||
<div className="text-right">
|
||||
<button
|
||||
type="submit"
|
||||
className="rounded bg-blue-500 px-4 py-2 text-white hover:bg-blue-600 focus:bg-blue-400"
|
||||
>
|
||||
Save
|
||||
</button>
|
||||
</div>
|
||||
</Form>
|
||||
);
|
||||
}
|
@ -1,70 +0,0 @@
|
||||
import type { LoaderArgs } from "@remix-run/node";
|
||||
import { json } from "@remix-run/node";
|
||||
import { Form, Link, NavLink, Outlet, useLoaderData } from "@remix-run/react";
|
||||
|
||||
import { getNoteListItems } from "~/models/note.server";
|
||||
import { requireUserId } from "~/session.server";
|
||||
import { useUser } from "~/utils";
|
||||
|
||||
export const loader = async ({ request }: LoaderArgs) => {
|
||||
const userId = await requireUserId(request);
|
||||
const noteListItems = await getNoteListItems({ userId });
|
||||
return json({ noteListItems });
|
||||
};
|
||||
|
||||
export default function NotesPage() {
|
||||
const data = useLoaderData<typeof loader>();
|
||||
const user = useUser();
|
||||
|
||||
return (
|
||||
<div className="flex h-full min-h-screen flex-col">
|
||||
<header className="flex items-center justify-between bg-slate-800 p-4 text-white">
|
||||
<h1 className="text-3xl font-bold">
|
||||
<Link to=".">Notes</Link>
|
||||
</h1>
|
||||
<p>{user.email}</p>
|
||||
<Form action="/logout" method="post">
|
||||
<button
|
||||
type="submit"
|
||||
className="rounded bg-slate-600 px-4 py-2 text-blue-100 hover:bg-blue-500 active:bg-blue-600"
|
||||
>
|
||||
Logout
|
||||
</button>
|
||||
</Form>
|
||||
</header>
|
||||
|
||||
<main className="flex h-full bg-white">
|
||||
<div className="h-full w-80 border-r bg-gray-50">
|
||||
<Link to="new" className="block p-4 text-xl text-blue-500">
|
||||
+ New Note
|
||||
</Link>
|
||||
|
||||
<hr />
|
||||
|
||||
{data.noteListItems.length === 0 ? (
|
||||
<p className="p-4">No notes yet</p>
|
||||
) : (
|
||||
<ol>
|
||||
{data.noteListItems.map((note) => (
|
||||
<li key={note.id}>
|
||||
<NavLink
|
||||
className={({ isActive }) =>
|
||||
`block border-b p-4 text-xl ${isActive ? "bg-white" : ""}`
|
||||
}
|
||||
to={note.id}
|
||||
>
|
||||
📝 {note.title}
|
||||
</NavLink>
|
||||
</li>
|
||||
))}
|
||||
</ol>
|
||||
)}
|
||||
</div>
|
||||
|
||||
<div className="flex-1 p-6">
|
||||
<Outlet />
|
||||
</div>
|
||||
</main>
|
||||
</div>
|
||||
);
|
||||
}
|
87
app/routes/sources.$id.tsx
Normal file
87
app/routes/sources.$id.tsx
Normal file
@ -0,0 +1,87 @@
|
||||
import type { ContentSource } from "@prisma/client";
|
||||
import type { ActionArgs, LoaderArgs } from "@remix-run/node";
|
||||
import { json, redirect } from "@remix-run/node";
|
||||
import { Link, useLoaderData } from "@remix-run/react";
|
||||
import { Breadcrumbs } from "~/components/breadcrumbs";
|
||||
import { getSource, saveSource } from "~/models/source.server";
|
||||
import { notFound } from "~/utils";
|
||||
|
||||
|
||||
export async function action({ request, params }: ActionArgs) {
|
||||
const formData = await request.formData();
|
||||
const id = formData.get("id") as string;
|
||||
const name = formData.get("name") as string;
|
||||
const type = formData.get("type") as string;
|
||||
const description = formData.get("description") as string;
|
||||
const connectionUrl = formData.get("connectionUrl") as string;
|
||||
await saveSource({ id, name, description, type, connectionUrl });
|
||||
return redirect("/sources");
|
||||
}
|
||||
|
||||
export async function loader({ params }: LoaderArgs) {
|
||||
const { id } = params;
|
||||
if (!id) {
|
||||
throw notFound();
|
||||
}
|
||||
const source = id !== "new" ? await getSource(id) : {
|
||||
type: "json"
|
||||
} as ContentSource;
|
||||
if (!source) {
|
||||
throw notFound();
|
||||
}
|
||||
return json({ source });
|
||||
}
|
||||
|
||||
|
||||
export default function SourcePage() {
|
||||
const { source } = useLoaderData<typeof loader>();
|
||||
|
||||
return (
|
||||
<>
|
||||
<Breadcrumbs>
|
||||
<Link to="/sources">Sources</Link>
|
||||
<Link to={`/sources/${source.id}`}>{source.name ?? "New Source"}</Link>
|
||||
</Breadcrumbs>
|
||||
<form method="post">
|
||||
<input type="hidden" name="id" value={source.id} />
|
||||
<div className="form-control w-full max-w-lg">
|
||||
<label className="label">
|
||||
<span className="label-text">Name</span>
|
||||
</label>
|
||||
<input type="text" name="name" placeholder="Type here"
|
||||
className="input input-bordered w-full"
|
||||
defaultValue={source.name} />
|
||||
</div>
|
||||
<div className="form-control w-full max-w-lg">
|
||||
<label className="label">
|
||||
<span className="label-text">Description</span>
|
||||
</label>
|
||||
<textarea name="description" placeholder="Type here"
|
||||
className="textarea textarea-bordered w-full"
|
||||
defaultValue={source.description!} />
|
||||
</div>
|
||||
<div className="form-control w-full max-w-lg">
|
||||
<label className="label">
|
||||
<span className="label-text">URL</span>
|
||||
</label>
|
||||
<input type="text" name="connectionUrl" placeholder="Type here"
|
||||
className="input input-bordered w-full"
|
||||
defaultValue={source.connectionUrl} />
|
||||
</div>
|
||||
<div className="form-control w-full max-w-lg">
|
||||
<label className="label">
|
||||
<span className="label-text">Type</span>
|
||||
</label>
|
||||
<input type="text" name="type" placeholder="Type here"
|
||||
className="input input-bordered w-full"
|
||||
readOnly
|
||||
defaultValue={source.type} />
|
||||
</div>
|
||||
<div className="form-control w-full max-w-lg mt-3">
|
||||
<button type="submit" className="btn btn-primary">Save</button>
|
||||
</div>
|
||||
|
||||
</form>
|
||||
</>
|
||||
);
|
||||
}
|
34
app/routes/sources._index.tsx
Normal file
34
app/routes/sources._index.tsx
Normal file
@ -0,0 +1,34 @@
|
||||
import { PlusSmallIcon } from "@heroicons/react/24/solid";
|
||||
import { json } from "@remix-run/node";
|
||||
import { Link, useLoaderData } from "@remix-run/react";
|
||||
import { Breadcrumbs } from "~/components/breadcrumbs";
|
||||
import { getSources } from "~/models/source.server";
|
||||
|
||||
export async function loader() {
|
||||
const sources = await getSources();
|
||||
|
||||
return json({ sources });
|
||||
}
|
||||
|
||||
export default function SourceIndex() {
|
||||
const { sources } = useLoaderData<typeof loader>();
|
||||
|
||||
return (
|
||||
<>
|
||||
<Breadcrumbs>
|
||||
<Link to="/sources">Sources</Link>
|
||||
<Link to="/sources/new">
|
||||
<PlusSmallIcon className="w-4 h-4 mr-2 stroke-current" />
|
||||
Add Source
|
||||
</Link>
|
||||
</Breadcrumbs>
|
||||
<ul className="menu bg-base-100 w-56">
|
||||
{sources.map(source => {
|
||||
return (
|
||||
<li key={source.id}><Link to={"/sources/" + source.id}>{source.name}</Link></li>
|
||||
);
|
||||
})}
|
||||
</ul>
|
||||
</>
|
||||
);
|
||||
}
|
7
app/routes/sources.tsx
Normal file
7
app/routes/sources.tsx
Normal file
@ -0,0 +1,7 @@
|
||||
import { Outlet } from "@remix-run/react";
|
||||
|
||||
export default function SourceLayout() {
|
||||
return (
|
||||
<Outlet />
|
||||
);
|
||||
}
|
89
app/utils.ts
89
app/utils.ts
@ -13,18 +13,18 @@ const DEFAULT_REDIRECT = "/";
|
||||
* @param {string} defaultRedirect The redirect to use if the to is unsafe.
|
||||
*/
|
||||
export function safeRedirect(
|
||||
to: FormDataEntryValue | string | null | undefined,
|
||||
defaultRedirect: string = DEFAULT_REDIRECT
|
||||
to: FormDataEntryValue | string | null | undefined,
|
||||
defaultRedirect: string = DEFAULT_REDIRECT
|
||||
) {
|
||||
if (!to || typeof to !== "string") {
|
||||
return defaultRedirect;
|
||||
}
|
||||
if (!to || typeof to !== "string") {
|
||||
return defaultRedirect;
|
||||
}
|
||||
|
||||
if (!to.startsWith("/") || to.startsWith("//")) {
|
||||
return defaultRedirect;
|
||||
}
|
||||
if (!to.startsWith("/") || to.startsWith("//")) {
|
||||
return defaultRedirect;
|
||||
}
|
||||
|
||||
return to;
|
||||
return to;
|
||||
}
|
||||
|
||||
/**
|
||||
@ -34,38 +34,67 @@ export function safeRedirect(
|
||||
* @returns {JSON|undefined} The router data or undefined if not found
|
||||
*/
|
||||
export function useMatchesData(
|
||||
id: string
|
||||
id: string
|
||||
): Record<string, unknown> | undefined {
|
||||
const matchingRoutes = useMatches();
|
||||
const route = useMemo(
|
||||
() => matchingRoutes.find((route) => route.id === id),
|
||||
[matchingRoutes, id]
|
||||
);
|
||||
return route?.data;
|
||||
const matchingRoutes = useMatches();
|
||||
const route = useMemo(
|
||||
() => matchingRoutes.find((route) => route.id === id),
|
||||
[matchingRoutes, id]
|
||||
);
|
||||
return route?.data;
|
||||
}
|
||||
|
||||
function isUser(user: any): user is User {
|
||||
return user && typeof user === "object" && typeof user.email === "string";
|
||||
return user && typeof user === "object" && typeof user.email === "string";
|
||||
}
|
||||
|
||||
export function useOptionalUser(): User | undefined {
|
||||
const data = useMatchesData("root");
|
||||
if (!data || !isUser(data.user)) {
|
||||
return undefined;
|
||||
}
|
||||
return data.user;
|
||||
const data = useMatchesData("root");
|
||||
if (!data || !isUser(data.user)) {
|
||||
return undefined;
|
||||
}
|
||||
return data.user;
|
||||
}
|
||||
|
||||
export function useUser(): User {
|
||||
const maybeUser = useOptionalUser();
|
||||
if (!maybeUser) {
|
||||
throw new Error(
|
||||
"No user found in root loader, but user is required by useUser. If user is optional, try useOptionalUser instead."
|
||||
);
|
||||
}
|
||||
return maybeUser;
|
||||
const maybeUser = useOptionalUser();
|
||||
if (!maybeUser) {
|
||||
throw new Error(
|
||||
"No user found in root loader, but user is required by useUser. If user is optional, try useOptionalUser instead."
|
||||
);
|
||||
}
|
||||
return maybeUser;
|
||||
}
|
||||
|
||||
export function validateEmail(email: unknown): email is string {
|
||||
return typeof email === "string" && email.length > 3 && email.includes("@");
|
||||
return typeof email === "string" && email.length > 3 && email.includes("@");
|
||||
}
|
||||
|
||||
export function notFound(message?: string) {
|
||||
return new Response(null, {
|
||||
status: 404,
|
||||
statusText: ["Not Found", message].filter(Boolean).join(": ")
|
||||
});
|
||||
}
|
||||
|
||||
export function createIndex<T>(records: T[], keyFn: (t: T) => string): Map<string, T> {
|
||||
return records.reduce((index, record) => {
|
||||
index.set(keyFn(record), record);
|
||||
return index;
|
||||
}, new Map<string, T>());
|
||||
}
|
||||
|
||||
export function slugify(string: string): string {
|
||||
const a = "àáâäæãåāăąçćčđďèéêëēėęěğǵḧîïíīįìıİłḿñńǹňôöòóœøōõőṕŕřßśšşșťțûüùúūǘůűųẃẍÿýžźż·/_,:;";
|
||||
const b = "aaaaaaaaaacccddeeeeeeeegghiiiiiiiilmnnnnoooooooooprrsssssttuuuuuuuuuwxyyzzz------";
|
||||
const p = new RegExp(a.split("").join("|"), "g");
|
||||
|
||||
return string.toString().toLowerCase()
|
||||
.replace(/\s+/g, "-") // Replace spaces with -
|
||||
.replace(p, c => b.charAt(a.indexOf(c))) // Replace special characters
|
||||
.replace(/&/g, "-and-") // Replace & with 'and'
|
||||
.replace(/[^\w\-]+/g, "") // Remove all non-word characters
|
||||
.replace(/\-\-+/g, "-") // Replace multiple - with single -
|
||||
.replace(/^-+/, "") // Trim - from start of text
|
||||
.replace(/-+$/, ""); // Trim - from end of text
|
||||
}
|
||||
|
@ -1,51 +1,26 @@
|
||||
import { faker } from "@faker-js/faker";
|
||||
|
||||
describe("smoke tests", () => {
|
||||
afterEach(() => {
|
||||
cy.cleanupUser();
|
||||
});
|
||||
afterEach(() => {
|
||||
cy.cleanupUser();
|
||||
});
|
||||
|
||||
it("should allow you to register and login", () => {
|
||||
const loginForm = {
|
||||
email: `${faker.internet.userName()}@example.com`,
|
||||
password: faker.internet.password(),
|
||||
};
|
||||
it("should allow you to register and login", () => {
|
||||
const loginForm = {
|
||||
email: `${faker.internet.userName()}@example.com`,
|
||||
password: faker.internet.password()
|
||||
};
|
||||
|
||||
cy.then(() => ({ email: loginForm.email })).as("user");
|
||||
cy.then(() => ({ email: loginForm.email })).as("user");
|
||||
|
||||
cy.visitAndCheck("/");
|
||||
cy.visitAndCheck("/");
|
||||
|
||||
cy.findByRole("link", { name: /sign up/i }).click();
|
||||
cy.findByRole("link", { name: /sign up/i }).click();
|
||||
|
||||
cy.findByRole("textbox", { name: /email/i }).type(loginForm.email);
|
||||
cy.findByLabelText(/password/i).type(loginForm.password);
|
||||
cy.findByRole("button", { name: /create account/i }).click();
|
||||
cy.findByRole("textbox", { name: /email/i }).type(loginForm.email);
|
||||
cy.findByLabelText(/password/i).type(loginForm.password);
|
||||
cy.findByRole("button", { name: /create account/i }).click();
|
||||
|
||||
cy.findByRole("link", { name: /notes/i }).click();
|
||||
cy.findByRole("button", { name: /logout/i }).click();
|
||||
cy.findByRole("link", { name: /log in/i });
|
||||
});
|
||||
});
|
||||
|
||||
it("should allow you to make a note", () => {
|
||||
const testNote = {
|
||||
title: faker.lorem.words(1),
|
||||
body: faker.lorem.sentences(1),
|
||||
};
|
||||
cy.login();
|
||||
|
||||
cy.visitAndCheck("/");
|
||||
|
||||
cy.findByRole("link", { name: /notes/i }).click();
|
||||
cy.findByText("No notes yet");
|
||||
|
||||
cy.findByRole("link", { name: /\+ new note/i }).click();
|
||||
|
||||
cy.findByRole("textbox", { name: /title/i }).type(testNote.title);
|
||||
cy.findByRole("textbox", { name: /body/i }).type(testNote.body);
|
||||
cy.findByRole("button", { name: /save/i }).click();
|
||||
|
||||
cy.findByRole("button", { name: /delete/i }).click();
|
||||
|
||||
cy.findByText("No notes yet");
|
||||
});
|
||||
});
|
||||
|
92
package-lock.json
generated
92
package-lock.json
generated
@ -12,6 +12,8 @@
|
||||
"@remix-run/node": "^1.16.0",
|
||||
"@remix-run/react": "^1.16.0",
|
||||
"@remix-run/serve": "^1.16.0",
|
||||
"@sindresorhus/slugify": "^2.2.0",
|
||||
"@tailwindcss/typography": "^0.5.9",
|
||||
"bcryptjs": "^2.4.3",
|
||||
"daisyui": "^2.51.6",
|
||||
"isbot": "^3.6.8",
|
||||
@ -3183,6 +3185,57 @@
|
||||
"url": "https://github.com/sindresorhus/is?sponsor=1"
|
||||
}
|
||||
},
|
||||
"node_modules/@sindresorhus/slugify": {
|
||||
"version": "2.2.0",
|
||||
"resolved": "https://registry.npmjs.org/@sindresorhus/slugify/-/slugify-2.2.0.tgz",
|
||||
"integrity": "sha512-9Vybc/qX8Kj6pxJaapjkFbiUJPk7MAkCh/GFCxIBnnsuYCFPIXKvnLidG8xlepht3i24L5XemUmGtrJ3UWrl6w==",
|
||||
"dependencies": {
|
||||
"@sindresorhus/transliterate": "^1.0.0",
|
||||
"escape-string-regexp": "^5.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=12"
|
||||
},
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/sindresorhus"
|
||||
}
|
||||
},
|
||||
"node_modules/@sindresorhus/slugify/node_modules/escape-string-regexp": {
|
||||
"version": "5.0.0",
|
||||
"resolved": "https://registry.npmjs.org/escape-string-regexp/-/escape-string-regexp-5.0.0.tgz",
|
||||
"integrity": "sha512-/veY75JbMK4j1yjvuUxuVsiS/hr/4iHs9FTT6cgTexxdE0Ly/glccBAkloH/DofkjRbZU3bnoj38mOmhkZ0lHw==",
|
||||
"engines": {
|
||||
"node": ">=12"
|
||||
},
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/sindresorhus"
|
||||
}
|
||||
},
|
||||
"node_modules/@sindresorhus/transliterate": {
|
||||
"version": "1.6.0",
|
||||
"resolved": "https://registry.npmjs.org/@sindresorhus/transliterate/-/transliterate-1.6.0.tgz",
|
||||
"integrity": "sha512-doH1gimEu3A46VX6aVxpHTeHrytJAG6HgdxntYnCFiIFHEM/ZGpG8KiZGBChchjQmG0XFIBL552kBTjVcMZXwQ==",
|
||||
"dependencies": {
|
||||
"escape-string-regexp": "^5.0.0"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=12"
|
||||
},
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/sindresorhus"
|
||||
}
|
||||
},
|
||||
"node_modules/@sindresorhus/transliterate/node_modules/escape-string-regexp": {
|
||||
"version": "5.0.0",
|
||||
"resolved": "https://registry.npmjs.org/escape-string-regexp/-/escape-string-regexp-5.0.0.tgz",
|
||||
"integrity": "sha512-/veY75JbMK4j1yjvuUxuVsiS/hr/4iHs9FTT6cgTexxdE0Ly/glccBAkloH/DofkjRbZU3bnoj38mOmhkZ0lHw==",
|
||||
"engines": {
|
||||
"node": ">=12"
|
||||
},
|
||||
"funding": {
|
||||
"url": "https://github.com/sponsors/sindresorhus"
|
||||
}
|
||||
},
|
||||
"node_modules/@szmarczak/http-timer": {
|
||||
"version": "4.0.6",
|
||||
"resolved": "https://registry.npmjs.org/@szmarczak/http-timer/-/http-timer-4.0.6.tgz",
|
||||
@ -3194,6 +3247,32 @@
|
||||
"node": ">=10"
|
||||
}
|
||||
},
|
||||
"node_modules/@tailwindcss/typography": {
|
||||
"version": "0.5.9",
|
||||
"resolved": "https://registry.npmjs.org/@tailwindcss/typography/-/typography-0.5.9.tgz",
|
||||
"integrity": "sha512-t8Sg3DyynFysV9f4JDOVISGsjazNb48AeIYQwcL+Bsq5uf4RYL75C1giZ43KISjeDGBaTN3Kxh7Xj/vRSMJUUg==",
|
||||
"dependencies": {
|
||||
"lodash.castarray": "^4.4.0",
|
||||
"lodash.isplainobject": "^4.0.6",
|
||||
"lodash.merge": "^4.6.2",
|
||||
"postcss-selector-parser": "6.0.10"
|
||||
},
|
||||
"peerDependencies": {
|
||||
"tailwindcss": ">=3.0.0 || insiders"
|
||||
}
|
||||
},
|
||||
"node_modules/@tailwindcss/typography/node_modules/postcss-selector-parser": {
|
||||
"version": "6.0.10",
|
||||
"resolved": "https://registry.npmjs.org/postcss-selector-parser/-/postcss-selector-parser-6.0.10.tgz",
|
||||
"integrity": "sha512-IQ7TZdoaqbT+LCpShg46jnZVlhWD2w6iQYAcYXfHARZ7X1t/UGhhceQDs5X0cGqKvYlHNOuv7Oa1xmb0oQuA3w==",
|
||||
"dependencies": {
|
||||
"cssesc": "^3.0.0",
|
||||
"util-deprecate": "^1.0.2"
|
||||
},
|
||||
"engines": {
|
||||
"node": ">=4"
|
||||
}
|
||||
},
|
||||
"node_modules/@testing-library/cypress": {
|
||||
"version": "9.0.0",
|
||||
"resolved": "https://registry.npmjs.org/@testing-library/cypress/-/cypress-9.0.0.tgz",
|
||||
@ -10158,16 +10237,25 @@
|
||||
"resolved": "https://registry.npmjs.org/lodash.camelcase/-/lodash.camelcase-4.3.0.tgz",
|
||||
"integrity": "sha512-TwuEnCnxbc3rAvhf/LbG7tJUDzhqXyFnv3dtzLOPgCG/hODL7WFnsbwktkD7yUV0RrreP/l1PALq/YSg6VvjlA=="
|
||||
},
|
||||
"node_modules/lodash.castarray": {
|
||||
"version": "4.4.0",
|
||||
"resolved": "https://registry.npmjs.org/lodash.castarray/-/lodash.castarray-4.4.0.tgz",
|
||||
"integrity": "sha512-aVx8ztPv7/2ULbArGJ2Y42bG1mEQ5mGjpdvrbJcJFU3TbYybe+QlLS4pst9zV52ymy2in1KpFPiZnAOATxD4+Q=="
|
||||
},
|
||||
"node_modules/lodash.debounce": {
|
||||
"version": "4.0.8",
|
||||
"resolved": "https://registry.npmjs.org/lodash.debounce/-/lodash.debounce-4.0.8.tgz",
|
||||
"integrity": "sha512-FT1yDzDYEoYWhnSGnpE/4Kj1fLZkDFyqRb7fNt6FdYOSxlUWAtp42Eh6Wb0rGIv/m9Bgo7x4GhQbm5Ys4SG5ow=="
|
||||
},
|
||||
"node_modules/lodash.isplainobject": {
|
||||
"version": "4.0.6",
|
||||
"resolved": "https://registry.npmjs.org/lodash.isplainobject/-/lodash.isplainobject-4.0.6.tgz",
|
||||
"integrity": "sha512-oSXzaWypCMHkPC3NvBEaPHf0KsA5mvPrOPgQWDsbg8n7orZ290M0BmC/jgRZ4vcJ6DTAhjrsSYgdsW/F+MFOBA=="
|
||||
},
|
||||
"node_modules/lodash.merge": {
|
||||
"version": "4.6.2",
|
||||
"resolved": "https://registry.npmjs.org/lodash.merge/-/lodash.merge-4.6.2.tgz",
|
||||
"integrity": "sha512-0KpjqXRVvrYyCsX1swR/XTK0va6VQkQM6MNo7PqW77ByjAhoARA8EfrP1N4+KlKj8YS0ZUCtRT/YUuhyYDujIQ==",
|
||||
"dev": true
|
||||
"integrity": "sha512-0KpjqXRVvrYyCsX1swR/XTK0va6VQkQM6MNo7PqW77ByjAhoARA8EfrP1N4+KlKj8YS0ZUCtRT/YUuhyYDujIQ=="
|
||||
},
|
||||
"node_modules/lodash.once": {
|
||||
"version": "4.1.1",
|
||||
|
@ -33,6 +33,8 @@
|
||||
"@remix-run/node": "^1.16.0",
|
||||
"@remix-run/react": "^1.16.0",
|
||||
"@remix-run/serve": "^1.16.0",
|
||||
"@sindresorhus/slugify": "^2.2.0",
|
||||
"@tailwindcss/typography": "^0.5.9",
|
||||
"bcryptjs": "^2.4.3",
|
||||
"daisyui": "^2.51.6",
|
||||
"isbot": "^3.6.8",
|
||||
|
@ -1,31 +0,0 @@
|
||||
-- CreateTable
|
||||
CREATE TABLE "User" (
|
||||
"id" TEXT NOT NULL PRIMARY KEY,
|
||||
"email" TEXT NOT NULL,
|
||||
"createdAt" DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
|
||||
"updatedAt" DATETIME NOT NULL
|
||||
);
|
||||
|
||||
-- CreateTable
|
||||
CREATE TABLE "Password" (
|
||||
"hash" TEXT NOT NULL,
|
||||
"userId" TEXT NOT NULL,
|
||||
CONSTRAINT "Password_userId_fkey" FOREIGN KEY ("userId") REFERENCES "User" ("id") ON DELETE CASCADE ON UPDATE CASCADE
|
||||
);
|
||||
|
||||
-- CreateTable
|
||||
CREATE TABLE "Note" (
|
||||
"id" TEXT NOT NULL PRIMARY KEY,
|
||||
"title" TEXT NOT NULL,
|
||||
"body" TEXT NOT NULL,
|
||||
"createdAt" DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
|
||||
"updatedAt" DATETIME NOT NULL,
|
||||
"userId" TEXT NOT NULL,
|
||||
CONSTRAINT "Note_userId_fkey" FOREIGN KEY ("userId") REFERENCES "User" ("id") ON DELETE CASCADE ON UPDATE CASCADE
|
||||
);
|
||||
|
||||
-- CreateIndex
|
||||
CREATE UNIQUE INDEX "User_email_key" ON "User"("email");
|
||||
|
||||
-- CreateIndex
|
||||
CREATE UNIQUE INDEX "Password_userId_key" ON "Password"("userId");
|
@ -0,0 +1,96 @@
|
||||
-- CreateTable
|
||||
CREATE TABLE "User"
|
||||
(
|
||||
"id" TEXT NOT NULL PRIMARY KEY,
|
||||
"email" TEXT NOT NULL,
|
||||
"role" TEXT NOT NULL DEFAULT 'user',
|
||||
"createdAt" DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
|
||||
"updatedAt" DATETIME NOT NULL
|
||||
);
|
||||
|
||||
-- CreateTable
|
||||
CREATE TABLE "Password"
|
||||
(
|
||||
"hash" TEXT NOT NULL,
|
||||
"userId" TEXT NOT NULL,
|
||||
CONSTRAINT "Password_userId_fkey" FOREIGN KEY ("userId") REFERENCES "User" ("id") ON DELETE CASCADE ON UPDATE CASCADE
|
||||
);
|
||||
|
||||
-- CreateTable
|
||||
CREATE TABLE "Station"
|
||||
(
|
||||
"id" TEXT NOT NULL PRIMARY KEY,
|
||||
"name" TEXT NOT NULL,
|
||||
"slug" TEXT NOT NULL,
|
||||
"description" TEXT,
|
||||
"imgUrl" TEXT NOT NULL,
|
||||
"streamUrl" TEXT NOT NULL,
|
||||
"reliability" REAL NOT NULL,
|
||||
"popularity" REAL NOT NULL,
|
||||
"createdAt" DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
|
||||
"updatedAt" DATETIME NOT NULL
|
||||
);
|
||||
|
||||
-- CreateTable
|
||||
CREATE TABLE "Tag"
|
||||
(
|
||||
"id" TEXT NOT NULL PRIMARY KEY,
|
||||
"name" TEXT NOT NULL,
|
||||
"slug" TEXT NOT NULL,
|
||||
"createdAt" DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
|
||||
"updatedAt" DATETIME NOT NULL
|
||||
);
|
||||
|
||||
-- CreateTable
|
||||
CREATE TABLE "StationTag"
|
||||
(
|
||||
"id" TEXT NOT NULL PRIMARY KEY,
|
||||
"stationId" TEXT NOT NULL,
|
||||
"tagId" TEXT NOT NULL,
|
||||
"createdAt" DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
|
||||
"updatedAt" DATETIME NOT NULL,
|
||||
CONSTRAINT "StationTag_stationId_fkey" FOREIGN KEY ("stationId") REFERENCES "Station" ("id") ON DELETE RESTRICT ON UPDATE CASCADE,
|
||||
CONSTRAINT "StationTag_tagId_fkey" FOREIGN KEY ("tagId") REFERENCES "Tag" ("id") ON DELETE RESTRICT ON UPDATE CASCADE
|
||||
);
|
||||
|
||||
-- CreateTable
|
||||
CREATE TABLE "UserFavoriteStation"
|
||||
(
|
||||
"id" TEXT NOT NULL PRIMARY KEY,
|
||||
"stationId" TEXT NOT NULL,
|
||||
"userId" TEXT NOT NULL,
|
||||
"createdAt" DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
|
||||
"updatedAt" DATETIME NOT NULL,
|
||||
CONSTRAINT "UserFavoriteStation_userId_fkey" FOREIGN KEY ("userId") REFERENCES "User" ("id") ON DELETE RESTRICT ON UPDATE CASCADE,
|
||||
CONSTRAINT "UserFavoriteStation_stationId_fkey" FOREIGN KEY ("stationId") REFERENCES "Station" ("id") ON DELETE RESTRICT ON UPDATE CASCADE
|
||||
);
|
||||
|
||||
-- CreateTable
|
||||
CREATE TABLE "ContentSource"
|
||||
(
|
||||
"id" TEXT NOT NULL PRIMARY KEY,
|
||||
"name" TEXT NOT NULL,
|
||||
"type" TEXT NOT NULL,
|
||||
"description" TEXT,
|
||||
"connectionUrl" TEXT NOT NULL,
|
||||
"createdAt" DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
|
||||
"updatedAt" DATETIME NOT NULL
|
||||
);
|
||||
|
||||
-- CreateIndex
|
||||
CREATE UNIQUE INDEX "User_email_key" ON "User" ("email");
|
||||
|
||||
-- CreateIndex
|
||||
CREATE UNIQUE INDEX "Password_userId_key" ON "Password" ("userId");
|
||||
|
||||
-- CreateIndex
|
||||
CREATE UNIQUE INDEX "Station_streamUrl_key" ON "Station" ("streamUrl");
|
||||
|
||||
-- CreateIndex
|
||||
CREATE UNIQUE INDEX "Tag_name_key" ON "Tag" ("name");
|
||||
|
||||
-- CreateIndex
|
||||
CREATE UNIQUE INDEX "Tag_slug_key" ON "Tag" ("slug");
|
||||
|
||||
-- CreateIndex
|
||||
CREATE UNIQUE INDEX "ContentSource_connectionUrl_key" ON "ContentSource" ("connectionUrl");
|
@ -1,38 +1,86 @@
|
||||
datasource db {
|
||||
provider = "sqlite"
|
||||
url = env("DATABASE_URL")
|
||||
provider = "sqlite"
|
||||
url = env("DATABASE_URL")
|
||||
}
|
||||
|
||||
generator client {
|
||||
provider = "prisma-client-js"
|
||||
provider = "prisma-client-js"
|
||||
}
|
||||
|
||||
model User {
|
||||
id String @id @default(cuid())
|
||||
email String @unique
|
||||
id String @id @default(cuid())
|
||||
email String @unique
|
||||
role String @default("user")
|
||||
|
||||
createdAt DateTime @default(now())
|
||||
updatedAt DateTime @updatedAt
|
||||
createdAt DateTime @default(now())
|
||||
updatedAt DateTime @updatedAt
|
||||
|
||||
password Password?
|
||||
notes Note[]
|
||||
password Password?
|
||||
favoriteStations UserFavoriteStation[]
|
||||
}
|
||||
|
||||
model Password {
|
||||
hash String
|
||||
hash String
|
||||
|
||||
user User @relation(fields: [userId], references: [id], onDelete: Cascade, onUpdate: Cascade)
|
||||
userId String @unique
|
||||
user User @relation(fields: [userId], references: [id], onDelete: Cascade, onUpdate: Cascade)
|
||||
userId String @unique
|
||||
}
|
||||
|
||||
model Note {
|
||||
id String @id @default(cuid())
|
||||
title String
|
||||
body String
|
||||
model Station {
|
||||
id String @id @default(cuid())
|
||||
name String
|
||||
slug String
|
||||
description String?
|
||||
imgUrl String
|
||||
streamUrl String @unique
|
||||
reliability Float
|
||||
popularity Float
|
||||
tags StationTag[]
|
||||
|
||||
createdAt DateTime @default(now())
|
||||
updatedAt DateTime @updatedAt
|
||||
|
||||
user User @relation(fields: [userId], references: [id], onDelete: Cascade, onUpdate: Cascade)
|
||||
userId String
|
||||
createdAt DateTime @default(now())
|
||||
updatedAt DateTime @updatedAt
|
||||
UserFavoriteStation UserFavoriteStation[]
|
||||
}
|
||||
|
||||
model Tag {
|
||||
id String @id @default(cuid())
|
||||
name String @unique
|
||||
slug String @unique
|
||||
stations StationTag[]
|
||||
|
||||
createdAt DateTime @default(now())
|
||||
updatedAt DateTime @updatedAt
|
||||
}
|
||||
|
||||
model StationTag {
|
||||
id String @id @default(cuid())
|
||||
station Station @relation(fields: [stationId], references: [id])
|
||||
tag Tag @relation(fields: [tagId], references: [id])
|
||||
stationId String
|
||||
tagId String
|
||||
|
||||
createdAt DateTime @default(now())
|
||||
updatedAt DateTime @updatedAt
|
||||
}
|
||||
|
||||
model UserFavoriteStation {
|
||||
id String @id @default(cuid())
|
||||
user User @relation(fields: [userId], references: [id])
|
||||
station Station @relation(fields: [stationId], references: [id])
|
||||
stationId String
|
||||
userId String
|
||||
|
||||
createdAt DateTime @default(now())
|
||||
updatedAt DateTime @updatedAt
|
||||
}
|
||||
|
||||
model ContentSource {
|
||||
id String @id @default(cuid())
|
||||
name String
|
||||
type String
|
||||
description String?
|
||||
connectionUrl String @unique
|
||||
|
||||
createdAt DateTime @default(now())
|
||||
updatedAt DateTime @updatedAt
|
||||
}
|
||||
|
@ -1,53 +1,58 @@
|
||||
import { PrismaClient } from "@prisma/client";
|
||||
import bcrypt from "bcryptjs";
|
||||
import { importSource } from "~/lib/importer.server";
|
||||
import { saveSource } from "~/models/source.server";
|
||||
|
||||
const prisma = new PrismaClient();
|
||||
|
||||
async function seed() {
|
||||
const email = "rachel@remix.run";
|
||||
const email = "testuser@foobar.com";
|
||||
|
||||
// cleanup the existing database
|
||||
await prisma.user.delete({ where: { email } }).catch(() => {
|
||||
// no worries if it doesn't exist yet
|
||||
});
|
||||
// cleanup the existing database
|
||||
await prisma.user.delete({ where: { email } }).catch(() => {
|
||||
// no worries if it doesn't exist yet
|
||||
});
|
||||
|
||||
const hashedPassword = await bcrypt.hash("racheliscool", 10);
|
||||
const hashedPassword = await bcrypt.hash("testuser", 10);
|
||||
|
||||
const user = await prisma.user.create({
|
||||
data: {
|
||||
email,
|
||||
password: {
|
||||
create: {
|
||||
hash: hashedPassword,
|
||||
},
|
||||
},
|
||||
},
|
||||
});
|
||||
await prisma.user.create({
|
||||
data: {
|
||||
email,
|
||||
password: {
|
||||
create: {
|
||||
hash: hashedPassword
|
||||
}
|
||||
}
|
||||
}
|
||||
});
|
||||
|
||||
await prisma.note.create({
|
||||
data: {
|
||||
title: "My first note",
|
||||
body: "Hello, world!",
|
||||
userId: user.id,
|
||||
},
|
||||
});
|
||||
const source = {
|
||||
name: "Take Home Challenge",
|
||||
connectionUrl: "https://s3-us-west-1.amazonaws.com/cdn-web.tunein.com/stations.json",
|
||||
type: "json"
|
||||
};
|
||||
|
||||
await prisma.note.create({
|
||||
data: {
|
||||
title: "My second note",
|
||||
body: "Hello, world!",
|
||||
userId: user.id,
|
||||
},
|
||||
});
|
||||
await prisma.contentSource
|
||||
.delete({
|
||||
where: { connectionUrl: source.connectionUrl }
|
||||
})
|
||||
.catch(() => {
|
||||
// no worries if it doesn't exist yet
|
||||
});
|
||||
|
||||
console.log(`Database has been seeded. 🌱`);
|
||||
const s = await saveSource(source);
|
||||
|
||||
await importSource(s);
|
||||
|
||||
|
||||
console.log(`Database has been seeded. 🌱`);
|
||||
}
|
||||
|
||||
seed()
|
||||
.catch((e) => {
|
||||
console.error(e);
|
||||
process.exit(1);
|
||||
})
|
||||
.finally(async () => {
|
||||
await prisma.$disconnect();
|
||||
});
|
||||
.catch((e) => {
|
||||
console.error(e);
|
||||
process.exit(1);
|
||||
})
|
||||
.finally(async () => {
|
||||
await prisma.$disconnect();
|
||||
});
|
||||
|
@ -1,13 +1,16 @@
|
||||
/** @type {import('@remix-run/dev').AppConfig} */
|
||||
/** @type {import("@remix-run/dev").AppConfig} */
|
||||
module.exports = {
|
||||
cacheDirectory: "./node_modules/.cache/remix",
|
||||
future: {
|
||||
v2_errorBoundary: true,
|
||||
v2_meta: true,
|
||||
v2_normalizeFormMethod: true,
|
||||
v2_routeConvention: true,
|
||||
},
|
||||
ignoredRouteFiles: ["**/.*", "**/*.test.{js,jsx,ts,tsx}"],
|
||||
postcss: true,
|
||||
tailwind: true,
|
||||
cacheDirectory: "./node_modules/.cache/remix",
|
||||
future: {
|
||||
v2_errorBoundary: true,
|
||||
v2_meta: true,
|
||||
v2_normalizeFormMethod: true,
|
||||
v2_routeConvention: true
|
||||
},
|
||||
ignoredRouteFiles: ["**/.*", "**/*.test.{js,jsx,ts,tsx}"],
|
||||
serverModuleFormat: "cjs",
|
||||
postcss: true,
|
||||
tailwind: true,
|
||||
sourcemap: true,
|
||||
serverDependenciesToBundle: []
|
||||
};
|
||||
|
@ -1,9 +1,9 @@
|
||||
import type { Config } from "tailwindcss";
|
||||
|
||||
export default {
|
||||
content: ["./app/**/*.{js,jsx,ts,tsx}"],
|
||||
theme: {
|
||||
extend: {}
|
||||
},
|
||||
plugins: [require("daisyui")]
|
||||
content: ["./app/**/*.{js,jsx,ts,tsx}"],
|
||||
theme: {
|
||||
extend: {}
|
||||
},
|
||||
plugins: [require("@tailwindcss/typography"), require("daisyui")]
|
||||
} satisfies Config;
|
||||
|
Loading…
x
Reference in New Issue
Block a user